内容目录
代码中常常会用到fmt.Sprintf
的格式化输出功能,这里做一个总结,方便以后查阅。
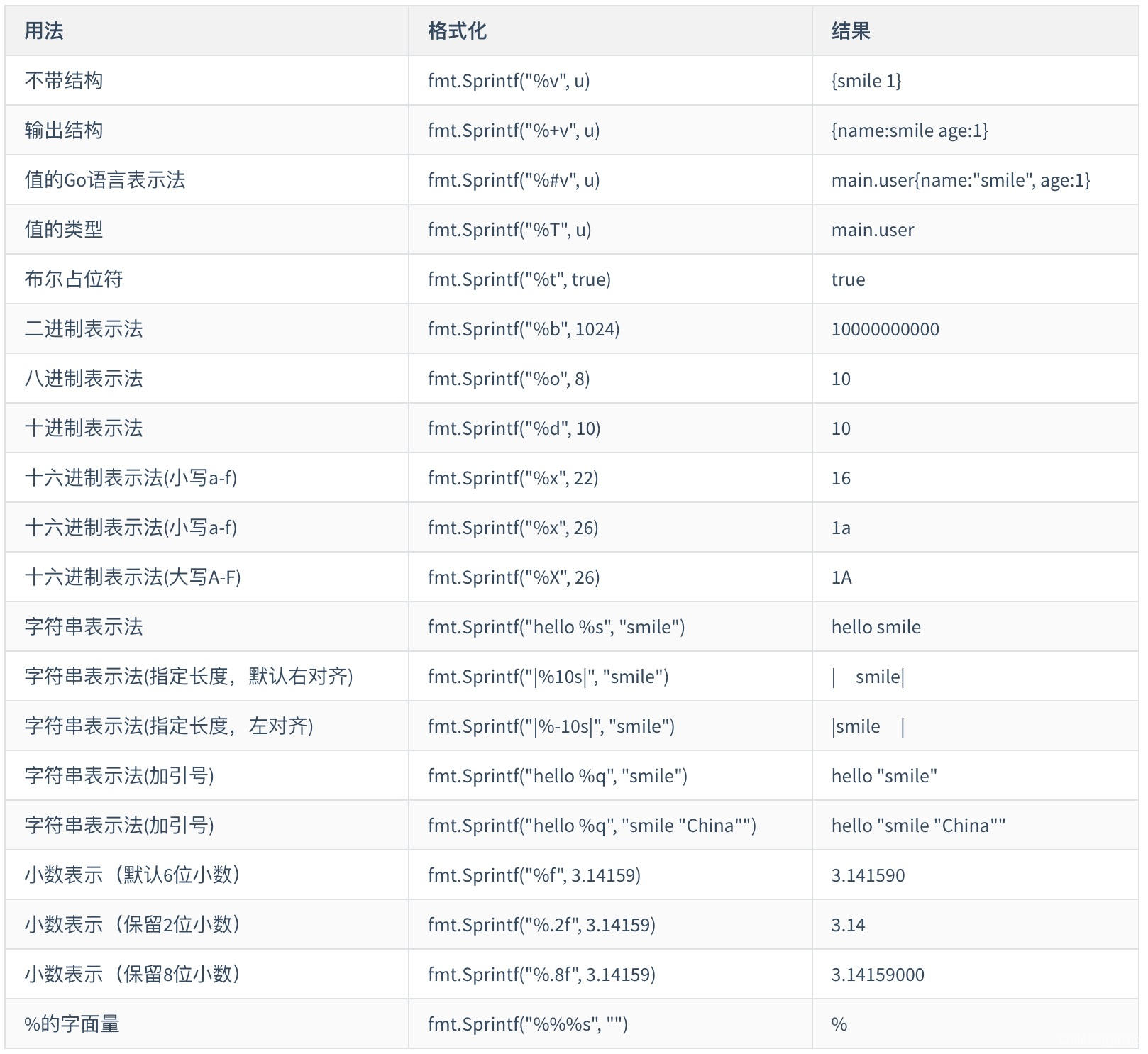
测试代码如下:
package main
import (
"fmt"
"strings"
"testing"
)
// TestSprintf 测试Printf 格式化输出
func TestSprintf(t *testing.T) {
type user struct {
name string
age int
}
u := user{"smile", 1}
tests := []struct {
name string // 测试名称
format string // 格式
value interface{} // 输入
want string // 期待的输出
}{
{"不带结构", "%v", u, `{smile 1}`}, // fmt.Sprintf("%v", u) == "{smile 1}"
{"输出结构", "%+v", u, `{name:smile age:1}`}, // 加号(+)会添加字段名输出
{"值的Go语言表示法", "%#v", u, `main.user{name:"smile", age:1}`},
{"值的类型", "%T", u, `main.user`},
{"布尔占位符", "%t", true, `true`}, // 参数只能是true 或者 false
{"二进制表示法", "%b", 1024, `10000000000`},
{"八进制表示法", "%o", 8, `10`},
{"十进制表示法", "%d", 10, `10`},
{"十六进制表示法(小写a-f)", "%x", 22, `16`},
{"十六进制表示法(小写a-f)", "%x", 26, `1a`},
{"十六进制表示法(大写A-F)", "%X", 26, `1A`},
{"字符串表示法", "hello %s", "smile", `hello smile`},
{"字符串表示法(指定长度,默认右对齐)", "|%10s|", "smile", `| smile|`},
{"字符串表示法(指定长度,左对齐)", "|%-10s|", "smile", `|smile |`},
{"字符串表示法(加引号)", "hello %q", "smile", `hello "smile"`},
{"字符串表示法(加引号)", "hello %q", `smile "China"`, `hello "smile \"China\""`},
{"小数表示(默认6位小数)", "%f", 3.14159, `3.141590`},
{"小数表示(保留2位小数)", "%.2f", 3.14159, `3.14`},
{"小数表示(保留8位小数)", "%.8f", 3.14159, `3.14159000`},
{"%的字面量", "%%%s", "", `%`},
}
results := make([]string, 0, len(tests))
for _, tt := range tests {
t.Run(tt.name, func(t *testing.T) {
got := fmt.Sprintf(tt.format, tt.value)
var result string
switch tt.value.(type) {
case string:
result = fmt.Sprintf("%s\t: fmt.Sprintf(%q, %q) = %q, want %q", tt.name, tt.format, tt.value, got, tt.want)
case user:
result = fmt.Sprintf("%s\t: fmt.Sprintf(%q, u) = %q, want %q", tt.name, tt.format, got, tt.want)
default:
result = fmt.Sprintf("%s\t: fmt.Sprintf(%q, %+v) = %q, want %q", tt.name, tt.format, tt.value, got, tt.want)
}
if got != tt.want {
t.Errorf(result)
} else {
results = append(results, result)
}
})
}
t.Cleanup(func() {
fmt.Println(strings.Join(results, "\n"))
})
}
0 条评论
撰写评论